Entity Framework 7 Getting Started
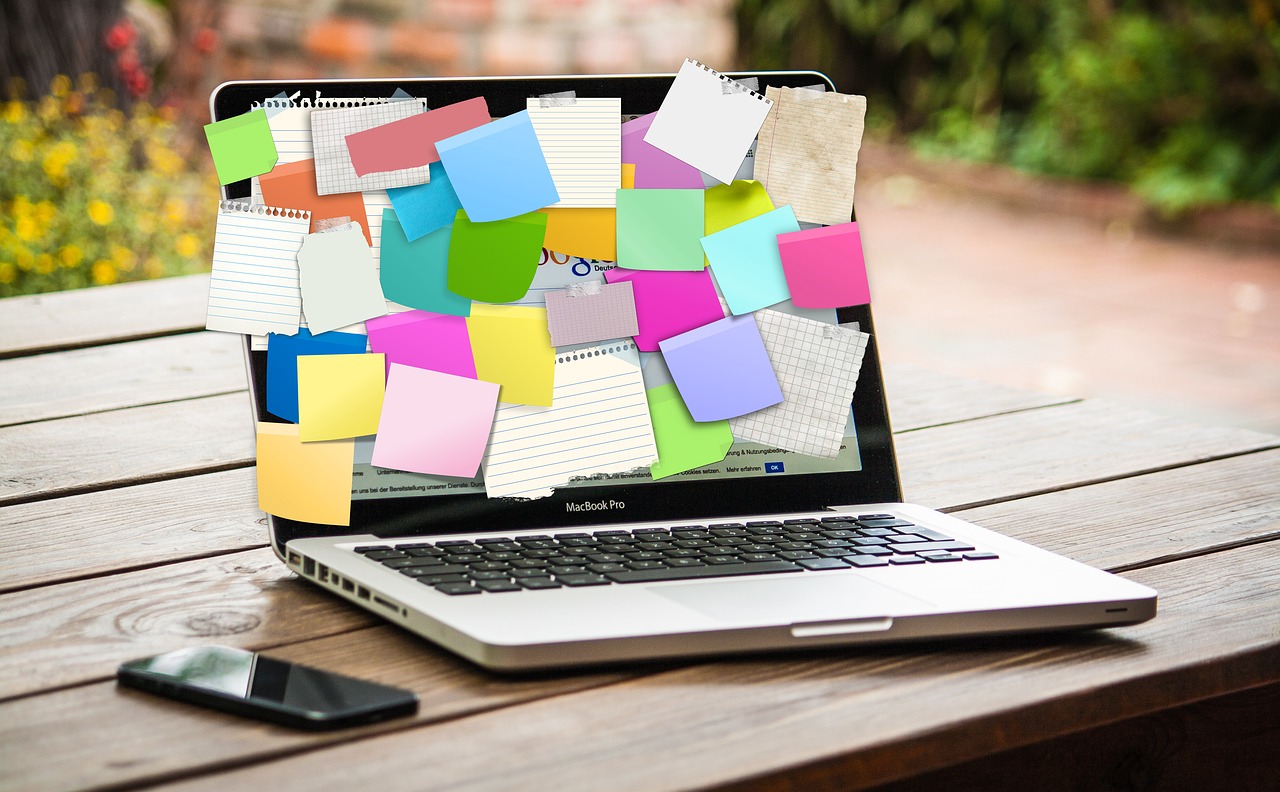
If you are new to Entity Framework, especially to all new EF7, best way to start is to look at this code from sample application. It shows all you need to know to get rolling: connecting to database, creating a table, inserting and selecting data all in one simple console app. You can run it in the new shiny Visual Studio 2015 with break points on any line that you want to verify and literally walk step by step understanding the process. Which is:
- Create entity class to represent the table (Blog).
- Create class that inherits from DbContext.
- Declare your entity in the DbContext.
- Register provider (UseSqlServer) and connection string as context options.
At this point your "back-end" is set and ready to go. To use it from the client:
- Use DbContext instance first making sure it creates database if not exists.
- Create entity object and add it to context.
- Save your changes with SaveChangesAsync.
- And finally query database with LINQ as usual.
You don't have to use async and without it code would be even simpler, but this is a good practice and doesn't add much complexity after all.
public class Program
{
public static async Task Main()
{
using (var db = new MyContext())
{
await db.Database.EnsureCreatedAsync();
}
using (var db = new MyContext())
{
var nextId = db.Blogs.Any() ? db.Blogs.Max(b => b.BlogId) + 1 : 1;
await db.AddAsync(new Blog { BlogId = nextId, Name = "Another Blog", Url = "http://example.com"
});
await db.SaveChangesAsync();
var blogs = db.Blogs.OrderBy(b => b.Name);
foreach (var item in blogs)
{
Console.WriteLine(item.Name);
}
}
}
}
public class MyContext : DbContext
{
public DbSet<Blog> Blogs { get; set; }
protected override void OnConfiguring(DbContextOptions builder)
{
builder.UseSqlServer(@"Server=(localdb)\v11.0;Database=Blogging;Trusted_Connection=True;");
}
}
public class Blog
{
public int BlogId { get; set; }
public string Name { get; set; }
public string Url { get; set; }
}
There are lots of other neat code in that sample app, so you might spend some time looking around. Next ASP.NET is still in the works, with very few documentation and tutorials available. In this situation looking at the code developers use themselves is very helpful.