Post Paging Extension
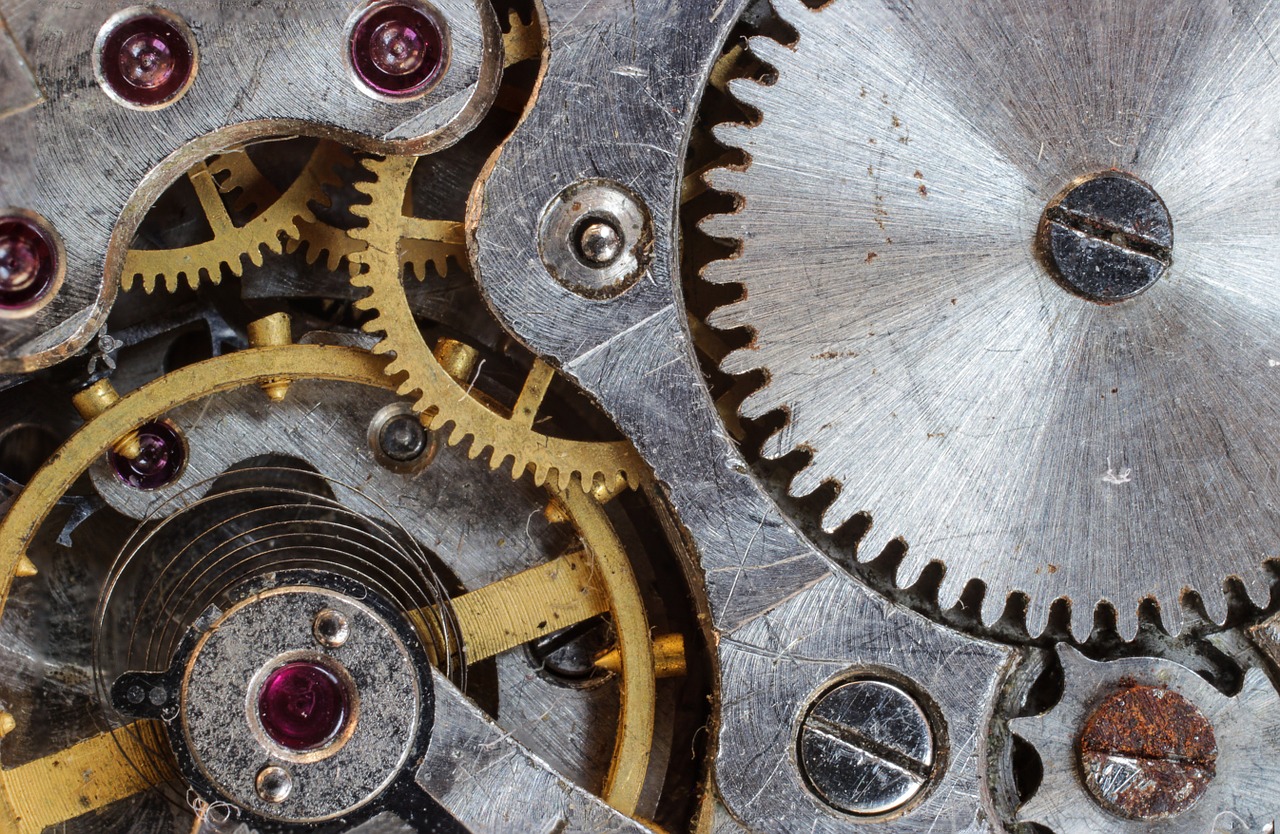
Reading this thread on Codeplex I was going to write that no such functionality exists but probably easy to add. But then I though just how easy it really would be? So I fired up Visual Studio and about half an hour later I got functioning plugin that does exactly what requested. And this is why I like BlogEngine - it is insanely easy to customize. At least, when you get used to it because documentation admittedly sucks.
What this extension does is it lets you split blog post into multiple pages by inserting page-break markers as shown in the picture below.
When you looking at the post list, you'll see first page with links to other pages.
After clicking link to the page two, you'll get to the single post with second page loaded, and clicking other links will reload page as expected, so visitor can navigate through the post topics.
I'm not necessarily thrilled with idea of hunting for the clicks, but this has valid and useful cases when you want represent content as several related topics and don't want to split them into separate posts.
And complete code is below, just in case you want to modify it or do something useful and need simple example. It also can be installed from BE gallery as compiled plugin using admin panel, as usual.
using System;
using System.Linq;
using System.Web;
using BlogEngine.Core;
using BlogEngine.Core.Web.Controls;
using BlogEngine.Core.Web.Extensions;
[Extension(
"Breaks a post into pages where [pagebreak] is found in the body and adds links to pages",
"3.1.1.0",
"<a href=\"http://www.rtur.net\">rtur.net</a>",
0)]
public class PostPaging
{
private static Post _post;
private static string _separator = "[pagebreak]";
private static string[] _pages;
static PostPaging()
{
Post.Serving += PostServing;
}
private static void PostServing(object sender, ServingEventArgs e)
{
if (!ExtensionManager.ExtensionEnabled("PostPaging"))
return;
if (!e.Body.Contains(_separator))
return;
_post = (Post)sender;
if(e.Location == ServingLocation.SinglePost || e.Location == ServingLocation.PostList)
{
BreakToPages(e);
}
else
{
e.Body = e.Body.Replace(_separator, "");
}
}
private static void BreakToPages(ServingEventArgs e)
{
string[] separator = new string[] { _separator };
_pages = e.Body.Split(separator, StringSplitOptions.RemoveEmptyEntries);
int iPage = 0;
var currentPage = HttpContext.Current.Request.QueryString["page"];
if (!string.IsNullOrEmpty(currentPage))
{
int.TryParse(currentPage, out iPage);
if (iPage > 0)
iPage--;
}
if (iPage >= _pages.Count())
HttpContext.Current.Response.Redirect("~/error404.aspx");
e.Body = _pages[iPage] + Pager(iPage);
}
private static string Pager(int current)
{
var links = "<div class=\"pagelinks\">Pages: ";
for (int i = 0; i < _pages.Count(); i++)
{
if (i == current)
{
links += string.Format("<span> {0} </span>", i + 1);
}
else
{
links += string.Format("<span> <a href=\"{0}?page={1}\">{1}</a> </span>", _post.RelativeLink, i + 1);
}
}
return links + "</div>";
}
}