Serializing XMLDocument to binary format
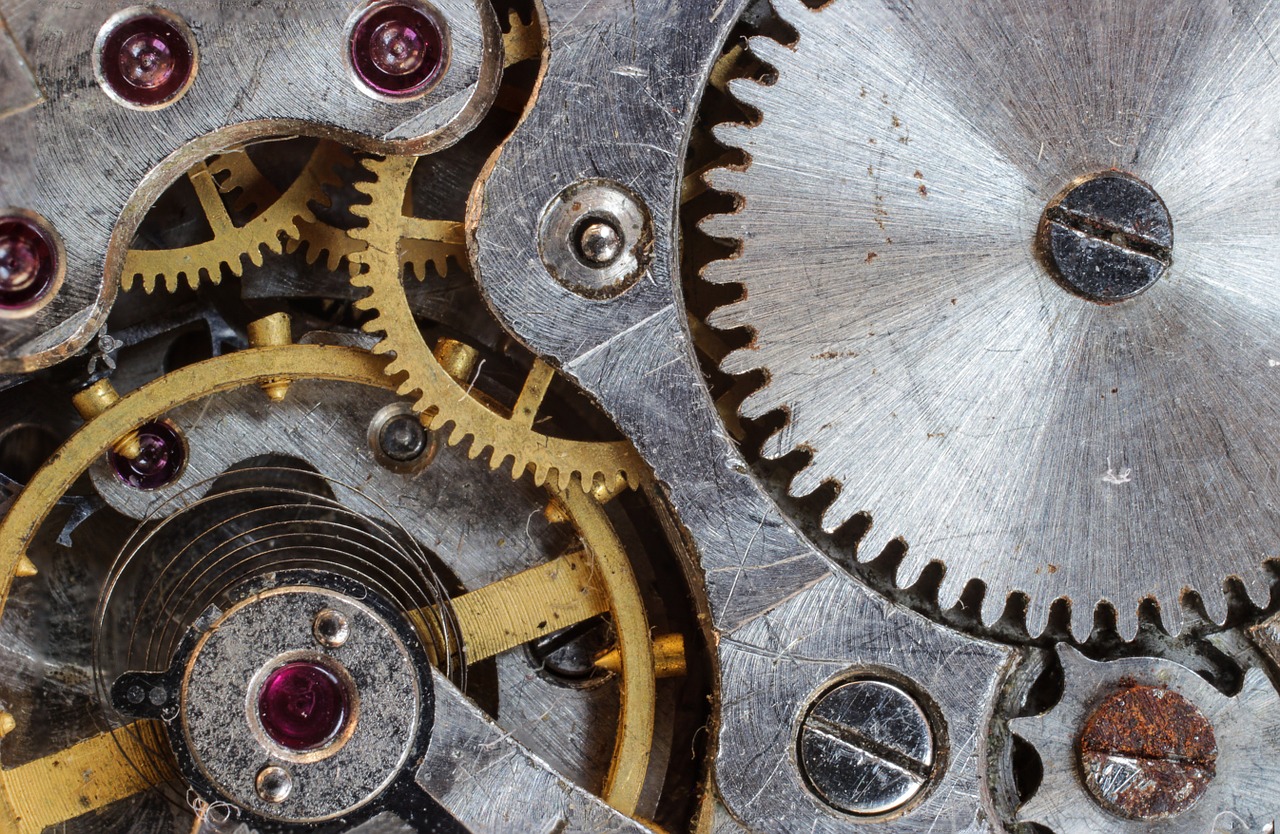
Why in the world would you want to do that? Well, for one, you might want to use it in Remoting. Second possibility is when you have different objects that you want to treat the same way, and XML Document is one of them. Whatever your situation is, XML Document is not serializable as is. You'll have to provide your own implementation - create class that inherits from XMLDocument and implements ISerializable interface:
[Serializable()]
public class CustomData : XmlDocument, ISerializable
// implementation here...
The truth is, you don't have to go all nine yards. If you think about it, XML document is really just a string with bunch of angle brackets in it. So instead of serializing document object, you can easily serialize this string - document's InnerXML.
[Serializable()]
public class CustomData
{
public CustomData() { }
private string innerXML = string.Empty;
public string InnerXML { get { return innerXML; } set { innerXML = value; } }
}
To serialize your document to binary, you'll do:
XMLDocument xml = GetDocument();
CustomData cd = new CustomData();
cd.InnerXML = xml.InnerXml;
// now you have serializable object
XmlSerializer x = new XmlSerializer(cd.GetType());
x.Serialize(writer, cd);
Deserializing is also straight forward:
BinaryFormatter bf = new BinaryFormatter();
CustomData cd = (CustomData)bf.Deserialize(stream);
XMLDocument xml = new XMLDocument();
xml.InnerXml = cd.InnerXML;
Now you have your XML document back, good as new and with all angle brackets you saved to binary stream. It may be a cheating, but it works and it is as simple as it gets.