Sorted GridView
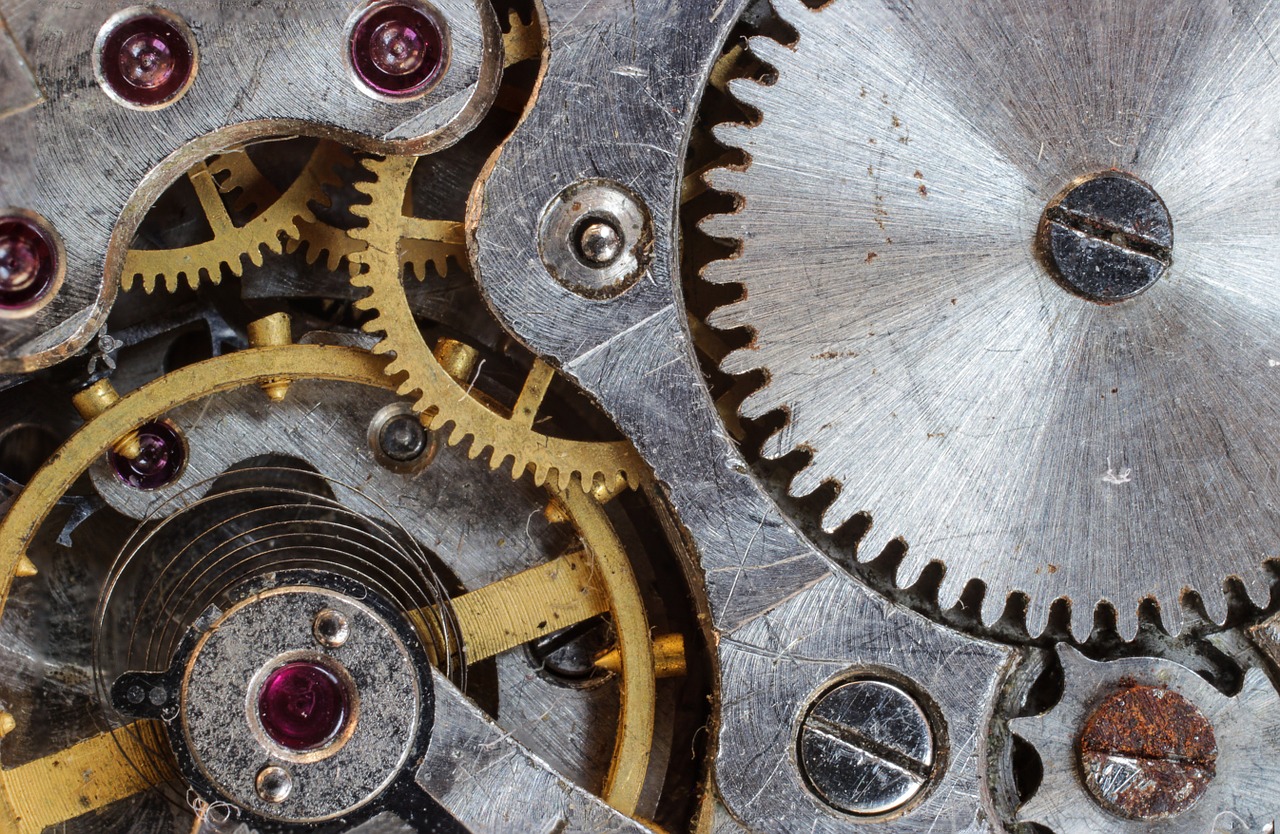
Did it ever happened to you? You have a problem and, after research and brainstorming, finally come up with this nice elegant solution. Two month (and hundred problems) later, you are staring at the screen with basically the same task in front of you. And trying to figure out - how the hell did you do it in the first place? Yes, I'm talking knowledge base - not an original idea and lots of people use their blogs exactly for this reason. I'm joining the crowd and will publish here code snippets under "code snippets" category. Here is the first one.
My task was to create sortable GridView, so I started with basic markup that has three data bound columns. Allow sorting set to true and OnSorting specifies function to run when column header is clicked.
<asp:GridView ID="grid"
runat="server"
AutoGenerateColumns="False"
AllowSorting="True"
OnSorting="gridView_Sorting" >
<Columns>
<asp:BoundField HeaderText="Download File" DataField="DownloadFile" SortExpression="DownloadFile" />
<asp:BoundField HeaderText="Download Date" DataField="DownloadDate" SortExpression="DownloadDate" />
<asp:BoundField HeaderText="User Agent" DataField="UserAgent" />
</Columns>
</asp:GridView>
Nice and easy, I got grid view, when click column header it gets sorted. One problem though: it always sorts ascending. Grid view does not remember that last time you clicked same column so it will not revert order for you. But you can ask to do just that if you keep track on column been clicked and sort direction. So, here two properties that will save itself into view state:
private string GridViewSortDirection
{
get { return ViewState["SortDirection"] as string ?? "ASC"; }
set { ViewState["SortDirection"] = value; }
}
private string GridViewSortExpression
{
get { return ViewState["SortExpression"] as string ?? string.Empty; }
set { ViewState["SortExpression"] = value; }
}
The sorting function knows what column was clicked, and this is pretty much all I'm interested in. Now, I need to check those properties to see if same column was clicked last time. If it is, I set sort direction to opposite of what saved and save new sorting order.
protected void gridView_Sorting(object sender, GridViewSortEventArgs e)
{
DataTable dataTable = grid.DataSource as DataTable;
if (dataTable != null)
{
DataView dataView = new DataView(dataTable);
dataView.Sort = GetSortExpression(e.SortExpression);
grid.DataSource = dataView;
grid.DataBind();
}
}
private string GetSortExpression(string sortExpression)
{
if (sortExpression == GridViewSortExpression && GridViewSortDirection == "ASC")
{
GridViewSortDirection = "DESC";
}
else
{
GridViewSortDirection = "ASC";
}
GridViewSortExpression = sortExpression;
return sortExpression + " " + GridViewSortDirection;
}
And here you have it: sorted grid view in few easy steps. If you want to see working example, you'll have to download DownloadCounter extension after I'm done with it, hopefully in the next couple days.