BlogEngine Widgets Tutorial
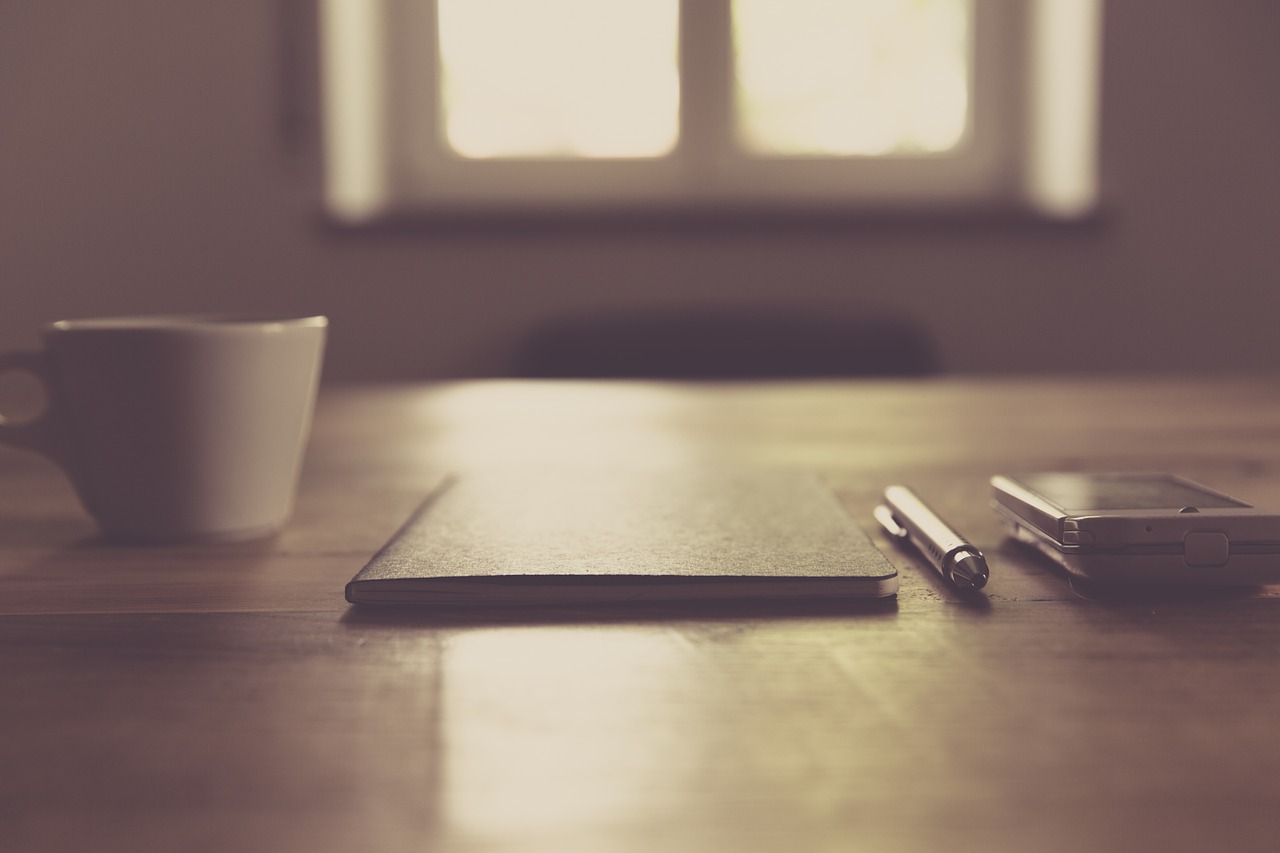
Widget is a special control you can add to the sidebar. What makes it special is that it is a member of the "zone" - area where you can add and configure these reusable components through the zone manager. It is similar to SharePoint WebParts - you can drag it around, set properties etc. And, in BlogEngine, you can build widget just in few simple steps.
In this tutorial we are going to build a widget to show selected Flickr photos in the sidebar using FlickrNet API. To make it work, all you need is download Flickr DLL (it also included in the .zip file in the end of this post) and drop it in the /bin folder. You also need at least BlogEngine 1.3.0.24 release. Now, lets build our widget.
Step one: plug it in
Open BlogEngine in Visual Studio (or Visual Web Developer) and add new folder under /widgets. Call it FlickrBar. In this folder create two user controls - widget.ascx and edit.ascx. First has to inherit from WidgetBase and second from WidgetEditBase, and entire code for both shown below.
public partial class widgets_FlickrBar_edit : WidgetEditBase
{
protected void Page_Load(object sender, EventArgs e) { }
public override void Save() { }
}
public partial class widgets_FlickrBar_widget : WidgetBase
{
protected void Page_Load(object sender, EventArgs e)
{
Name = "FlickrBar";
Title = "Flickr Photos";
}
}
Now you can log into your site and add newly created FlickrBar to the sidebar. It doesn't do anything useful yet, but it will show up with a title and edit/delete control buttons, you can drag it anywhere within the zone and it will behave just as any other widget. That's a start!
Step two: show time
What our little widget going to do is quiet simple: we want it run query against Flickr web service and show results as a mosaic of thumbnails that you can click on to navigate to the photo page if you find it interesting.
With FlickrNet library this is a snap. Entire code is shown below, and it is pretty self-explanatory. We create Flickr object, set some search options and call PhotosSearch method that returns collection of photos. Loop through collection and output images using StringBuilder - standard stuff. As a result, you should see in the sidebar something similar to the picture on the right.
The application key is something that you need to request from Flickr site when create your application, so I requested one and you can use it for this application freely.
using System;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Collections.Specialized;
using System.Text;
using FlickrNet;
public partial class widgets_FlickrBar_widget : WidgetBase
{
protected void Page_Load(object sender, EventArgs e)
{
Name = "FlickrBar";
Title = "Flickr Photos";
if (!Page.IsPostBack)
{
LoadPhotos();
}
}
private void LoadPhotos()
{
try
{
StringBuilder sb = new StringBuilder();
sb.Append("<div style=\"width:230px\">");
Flickr flickr = new Flickr("69e0627f9548359209a18d900cd4e666");
Flickr.CacheDisabled = true;
PhotoSearchOptions options = new PhotoSearchOptions();
options.PerPage = 9;
options.Page = 1;
options.Tags = "blue,sky";
Photos photos = flickr.PhotosSearch(options);
if (photos.PhotoCollection == null)
sb.Append("No photos available.");
else
{
foreach (Photo photo in photos.PhotoCollection)
{
sb.AppendFormat("<a href=\"{0}\"><img src=\"{1}\" title=\"{2}\"/></a>",
photo.WebUrl,
photo.SquareThumbnailUrl,
photo.Title);
}
}
sb.Append("</div>");
litFlickrPhotos.Text = sb.ToString();
}
catch (Exception) { }
}
}
Step three: customize it
You can stop right there, but of course it would be no fun if you can't customize your search options and look and feel of the widget. We can easily add this functionality and let blogger customize widget to fit his or her needs.
Just add input controls to the edit page and do load and save in the code behind as shown in the code snippet below. The only not standard technique there is using widgets settings to load and save data. It uses StringDictionary – couldn’t be simpler. And it saves it in the XML file or database according to your data provider and does caching for you.
public partial class widgets_FlickrBar_edit : WidgetEditBase
{
protected void Page_Load(object sender, EventArgs e)
{
StringDictionary sd = (StringDictionary)GetSettings(ObjectType.StringDictionary);
txtAppKey.Text = sd["AppKey"];
// more settings...
}
public override void Save()
{
StringDictionary sd = new StringDictionary();
sd.Add("AppKey", txtAppKey.Text);
// more settings...
base.SaveSettings(sd);
}
}
Please note that Widget framework is not a part of BlogEngine 1.3, it is coming in 1.4 and you can only use it with current SVN builds. Which means the purpose of this tutorial is to show some new functionality and chance for you to give your opinion. The code for this tutorial will only work for BlogEngine 1.3.0.24 and up. It is a tutorial code – it meant to be simple and limited in functionality. You are free to extend it and build a production quality widget or use it in any way you wish. Check out Flickr API examples and BlogEngine.Net Flickr Control for additional references.
Updated for versions 1.6.0 and 1.6.1