Doing Ajax using client callbacks
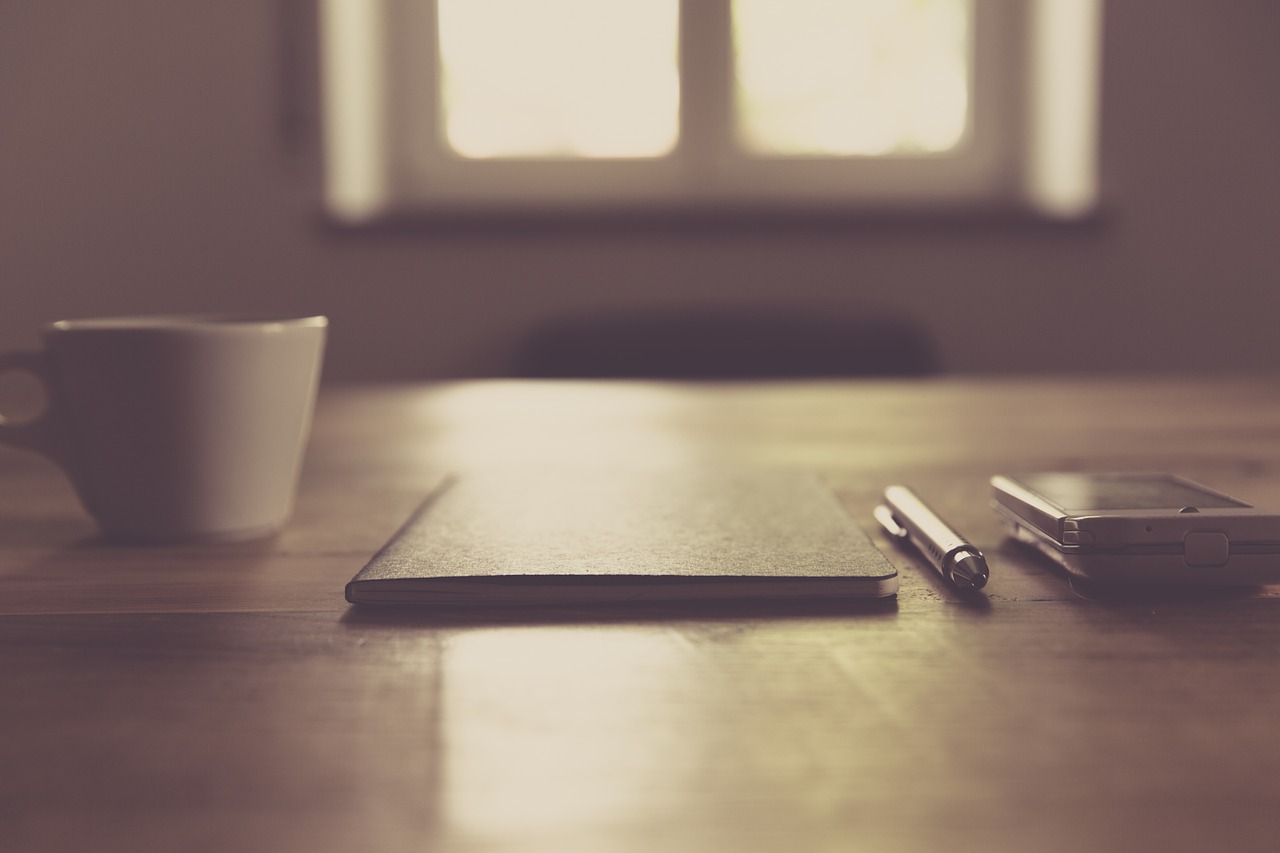
Yes, I've heard about Ajax before - one would have to be hiding in the hole for the last year or two to avoid the buzz. I've read articles, seen videos and presentations, even used applications that utilize Ajax on a daily basis. So I’m not exactly a newbie. But somehow I managed to stay away from it - no projects I've been involved into for the last few years used Ajax. I decided it is a shame and I want to change it. Here is a plan: for starters I'll write two small applications ("gadgets", or user controls, for BlogEngine). The first one will be using "classic" JavaScript callback approach and the other one will be doing similar stuff the Microsoft way. Then I will compare experience. Sounds fair?
I don't want to get lost into details doing these first steps, so applications should be pretty basic. But I don't want to waste my time either - so they got to be useful. The first one will be simple updatable panel (div). Because this is going to be a user control, I went to VS, created new folder under “~/User controls” and added .ascx file for this control. It has two “div” elements, one for input/editing and another to display data (see the first picture). Then I added .aspx file – the one that I’m going to use as “web service” for back end processing. It will parse and write data to the file, and then return data back to the JavaScript that invoked it. It would be better to use real web service instead of page, but I want it to be easily distributable as BlogEngine plug-in, or gadget or whatever this will be called, and page does not have any deployment overhead unlike alternatives, so I stick with the page until someone convince me otherwise.
Now, when we have user control and “web service” set up, here is how it should work: you add control to the side bar in the theme, on load it looks at the data directory to see if any data associated with this control saved and, if any, displays it in the div (pic.2 - no data yet). When you log in as admin, this div becomes clickable, and when you click it replaced by text area where you can type any HTML you like (pic.3). Editor panel also has a button so you can save your changes - and this is where Ajax will do its magic. On button click we will run JavaScript function; this function will use some of the Ajax functions that BlogEngine provides and will submit text area to the .aspx page. Code behind in the .aspx page will do back-end job, call back, JavaScript will update div and collapse editing area so things get back to normal (pic.4). Easy enough.
Here how you add control to the theme (I used SidePanel.ascx but you can add it anywhere, doesn’t matter). First, register control:
<%@ Register src="~/User Controls/SBPanel/SideBarPanel.ascx" TagName="SBPanel" TagPrefix="uc2" %>
Then, add it to the theme:
<div class="box">
<h1>Ajax callback example</h1>
<uc2:SBPanelID="sbpanel1"runat="server"/>
</div>
And, the key point in the whole story, there how you use Ajax the BlogEngine’s way – calling CreateCallback from the core framework. All you need to do is spesify page (or web service) you want to call and pass name of the JavaScript function you want to be called after server is done. You might want to take a look at BlogEngine.Core/Web/Scripts/blog.js – this is where CreateCallback coming from, along with dollar sign ($) short-cut function and some others. They all available to you at all times in BlogEngine client side code, because blog.js added to every page automatically by the framework.
function SBPanelSave()
{
txt = $("txtSBPanel").value;
file = "/BlogEngine.NET/User controls/SBPanel/SideBarPanel.aspx";
CreateCallback(file + "?txt=" + txt, SBPanelCallback);
}
In our call back, we take parsed and formatted by server data and update region of the page to display it, but it is up to you what to do in the call back function, you can do nothing if it make sense:
function SBPanelCallback(s)
{
panel = $("SBPanelShowAreaText");
panel.innerHTML = s;
HideSBPanelEdit();
}
User control discussed in this post you can download using link below. Let me tell you up front: it is NOT a production quality code; it is only proof of concept. I will turn it into gadget later on and make it available for download, but you can easily use it as is right now if you see a fit.
To get it working:
- Unzip folder that contains control files and change call to the .aspx page in the SideBarPanel.ascx to reflect path to your server (for example, in my case it would be http://rtur.net/blog/User controls/SBPanel/SideBarPanel.aspx).
- Upload folder and files to the host server. You should have directory structure as shown in the picture on the right.
- Register control in the ~/themes/standard/SidePanel.ascx the way described above (I only tested it with standard theme).
- Add control to HTML markup, again as was described in the text above (I appended it to the end of SidePanel.ascx).
Go to your website, click in the panel and type in some text. Click “Done” and you should see changes in you blog’s side bar.
Don’t get too creative with HTML you type in: the code uses “poor men’s” URL encoding, simply replacing angle brackets with square ones in the background.