Simple Widget Tutorial
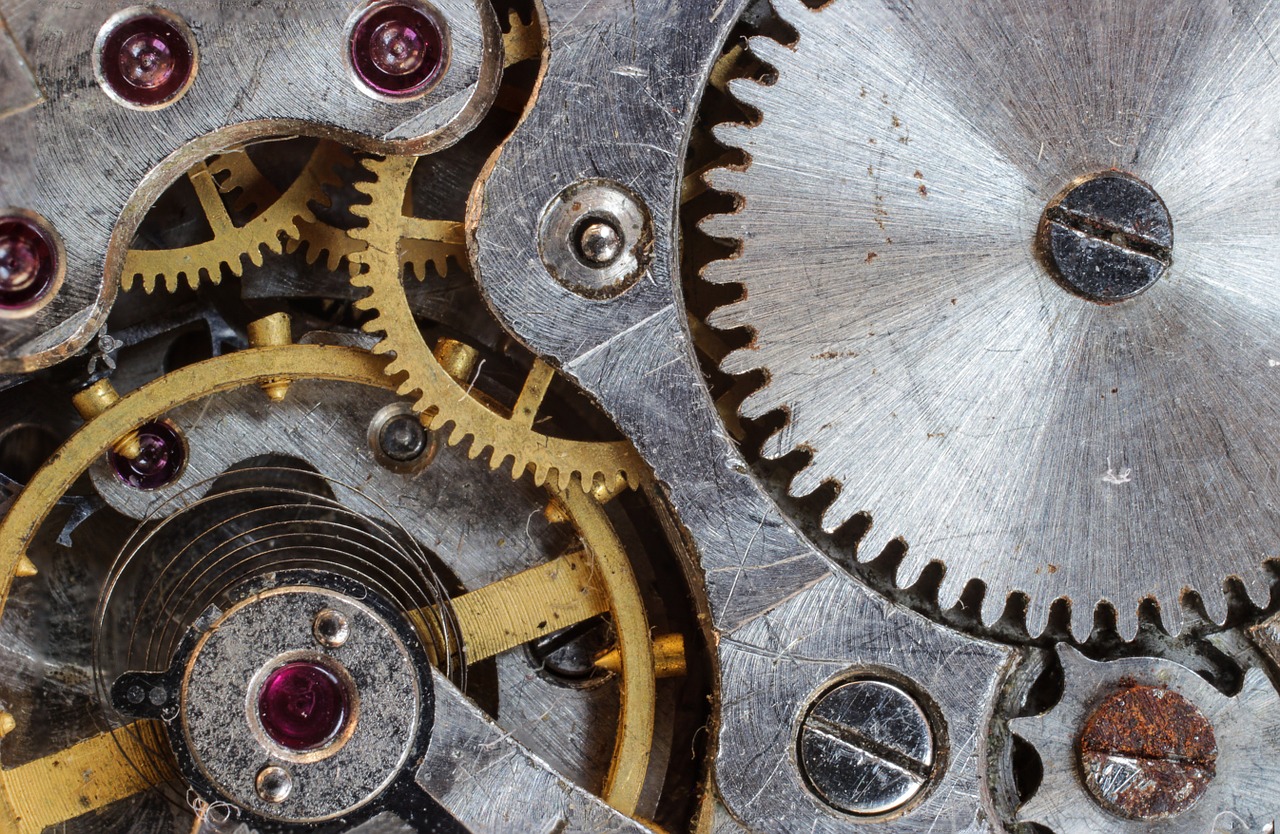
Feedjit is a service that provides live traffic feed for your site. It is easy to set up – you copy chunk of HTML and insert it into your blog’s markup. That is, if you know HTML and used to editing files in your blog, which shouldn’t be a requirement for average blogger. This is why popular blog providers supply Feedjit widgets – so that blogger does not have to edit files by hand and FTP them to the host. In this little exercise we create such a widget for BlogEngine.
Let’s start with basics. Open source project and add new folder: ~/widgets/Feedjit. Create new user control: ~/widgets/Feedjit/widget.ascx (with corresponding code behind file). Complete code for both classes shown below.
<%@ Control Language="C#" AutoEventWireup="true" CodeFile="widget.ascx.cs" Inherits="widgets_feedjit_widget" %>
<div style="text-align:center">
<script type="text/javascript" src="http://feedjit.com/serve/?bc=FFFFFF&amp;tc=494949&amp;brd1=FFFFFF&amp;lnk=69A22B&amp;hc=1A0D0D&amp;ww=210;"></script>
<noscript><a href="http://feedjit.com/">Feedjit Live Blog Stats</a></noscript>
</div>
using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class widgets_feedjit_widget : WidgetBase
{
public override string Name
{
get { return "Feedjit"; }
}
public override bool IsEditable
{
get { return false; }
}
public override void LoadWidget()
{
// nothing to load
}
}
If you start your blog, you should see new widget available in the widgets zone - “Feedjit”. Add it to the sidebar as any other standard BE.NET widget and you should see something similar to this picture. Web service will replace markup with information on latest visitors for your site. Very cool and easy, and it is customizable too – you can change font colors, links, borders, set widget width etc. This time by modifying query string parameters in the same chunk of code, either on Feedjit site or by hand. Lets eliminate this manual step and give our blogger nice easy form to input all those customizable values.
For this to happen, we need to create another control: ~/widgets/Feedjit/edit.ascx (again with code behind). This new control will provide edit capability to the widget and let us create that form where blogger can enter and change values. When we finished, it should look like one in the picture on the left. The code for “edit” control and it’s code behind both shown below.
<%@ Control Language="C#" AutoEventWireup="true" CodeFile="edit.ascx.cs" Inherits="widgets_Feedjit_edit" %>
<fieldset>
<legend>Feedjit Settings</legend>
<table>
<tr>
<td><label for="<%=txtHC.ClientID %>">Header Color</label></td>
<td><asp:TextBox runat="server" ID="txtHC" /></td>
</tr>
<tr>
<td><label for="<%=txtTC.ClientID %>">Text Color</label></td>
<td><asp:TextBox runat="server" ID="txtTC" /></td>
</tr>
<tr>
<td><label for="<%=txtLNK.ClientID %>">Link Color</label></td>
<td><asp:TextBox runat="server" ID="txtLNK" /></td>
</tr>
<tr>
<td><label for="<%=txtBRD1.ClientID %>">Border Color</label></td>
<td><asp:TextBox runat="server" ID="txtBRD1" /></td>
</tr>
<tr>
<td><label for="<%=txtBC.ClientID %>">Background Color</label></td>
<td><asp:TextBox runat="server" ID="txtBC" /></td>
</tr>
<tr>
<td><label for="<%=txtWW.ClientID %>">Width</label></td>
<td><asp:TextBox runat="server" ID="txtWW" /></td>
</tr>
</table>
</fieldset>
using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Collections.Specialized;
using BlogEngine.Core;
public partial class widgets_Feedjit_edit : WidgetEditBase
{
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
StringDictionary settings = GetSettings();
txtBC.Text = settings["BC"];
txtWW.Text = settings["WW"];
txtBRD1.Text = settings["BRD1"];
txtHC.Text = settings["HC"];
txtLNK.Text = settings["LNK"];
txtTC.Text = settings["TC"];
}
}
public override void Save()
{
StringDictionary settings = GetSettings();
settings["BC"] = txtBC.Text;
settings["WW"] = txtWW.Text;
settings["BRD1"] = txtBRD1.Text;
settings["HC"] = txtHC.Text;
settings["LNK"] = txtLNK.Text;
settings["TC"] = txtTC.Text;
SaveSettings(settings);
}
}
Now we need to make couple changes in the first widget control so that it will use customized values in place of hard-coded string we are copied on the Feedjit’s web site. First, we need to change “IsEditable” property to “true”, and then add a getter that will build and return query string dynamically.
public string FeedjitQS
{
get
{
string tmpl = "http://feedjit.com/serve/?bc={0}&amp;tc={1}&amp;brd1={2}&amp;lnk={3}&amp;hc={4}&amp;ww={5};";
StringDictionary s = GetSettings();
return string.Format(tmpl, s["BC"], s["TC"], s["BRD1"], s["LNK"], s["HC"], s["WW"]);
}
}
And now we just replace java script source with one built in the previous step:
<%@ Control Language="C#" AutoEventWireup="true" CodeFile="widget.ascx.cs" Inherits="widgets_Feedjit_widget" %>
<div style="text-align:center">
<script type="text/javascript" src=<%=FeedjitQS%>></script>
<noscript><a href="http://feedjit.com/">Feedjit Live Blog Stats</a></noscript>
</div>
That should do it. Run your project and you will be able to load edit form by clicking “edit” button on the widget. Blogger using your widget can change look an feel of the Feedjit on the fly without ever touching any code. The only thing missing in BE.NET is automated widget installer, so for now you still have to do usual unzip/ftp files to your site. But I’m sure it will change in the near future.