Tutorial - Building NivoSlider Extension (Part 1)
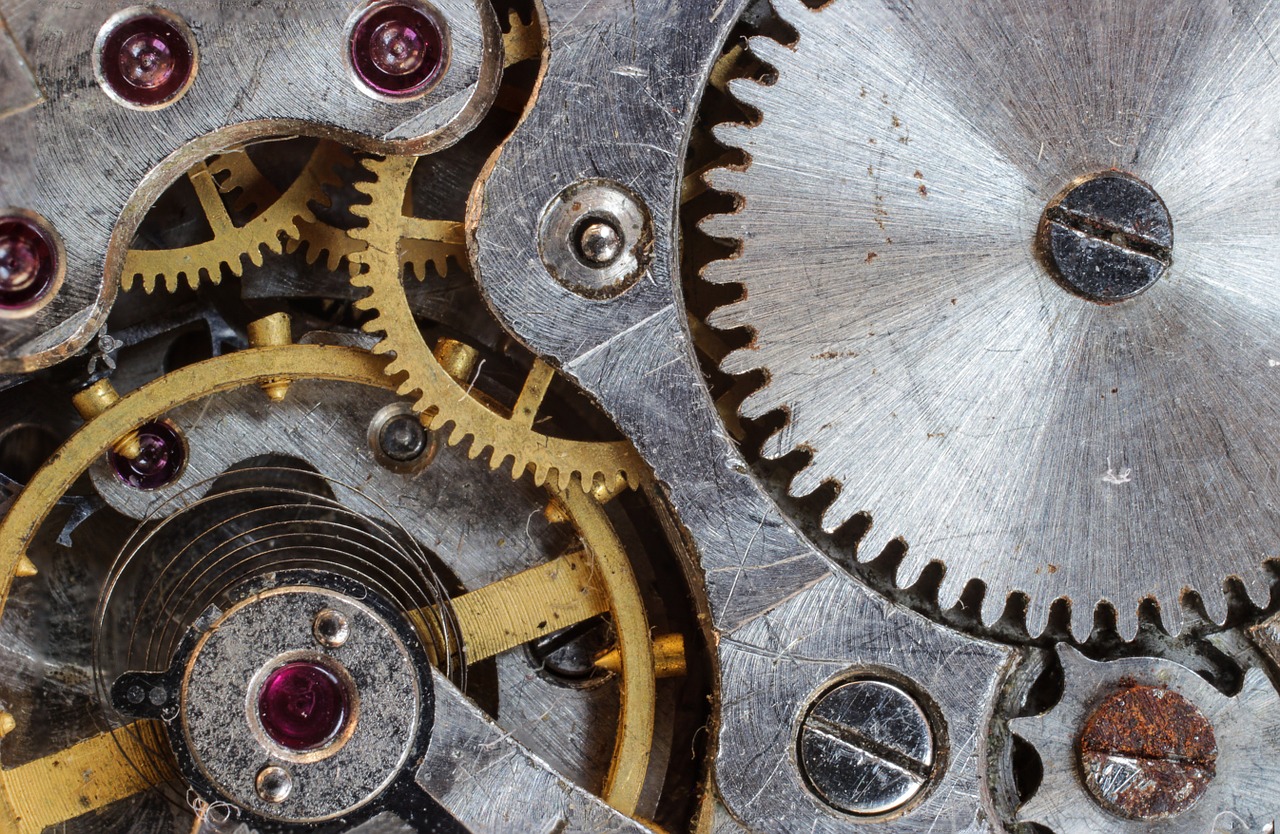
Getting Started - Pure HTML Code
<link rel="stylesheet" type="text/css" href="http://www.rtur.net/blog/Custom/Extensions/NivoSlider/nivo-slider.css">
<script type="text/javascript" src="/blog/Custom/Extensions/NivoSlider/jquery.nivo.slider.pack.js"></script>
<script type="text/javascript">$(window).load(function (){ LoadSlider('#slider1');});</script>
When I built theme for this site I used excellent Boldy theme as a template, and original theme has nice jQuery slider for a front page. I did not need it at the time, but later used it for another project and liked how simple and light-weight this slider is. In this tutorial we’ll transform NivoSlider into full-featured extension for BlogEngine.NET 2.5 and learn few tips and tricks along the way that will help you add useful functionality to your blog with no sweat.
To integrate component that uses another technology into your web application, first thing you need to understand is how it works client-side only so you can isolate pieces required by your project without PHP or whatever server-side code that component uses. Luckily, NivoSlider can be downloaded as stand-alone jQuery plugin (free under MIT license) with working plain HTML demo, so we don’t even have to do any investigative work figuring out what HTML/JavaScrip/CSS code we need to extract. Striped down for readability version looks something like this:
<div id="slider" class="nivoSlider">
<a href="#"><img src="images/toystory.jpg" alt="" /></a>
<a href="#"><img src="images/up.jpg" alt="" title="This is a caption" /></a>
</div>
<script type="text/javascript" src="scripts/jquery-1.6.1.min.js"></script>
<script type="text/javascript" src="../jquery.nivo.slider.pack.js"></script>
<script type="text/javascript">
$(window).load(function() { $('#slider').nivoSlider(); });
</script>
As you can see, on window load jQuery will attach nivoSlider() function to element with ID slider
, and using this handler jQuery (add-on to be precise) will run the show.
Moving To Server Side
BlogEngine comes with jQuery pre-installed so we only need nivo.slider.pack add-on plus miscellanies CSS/image files, nothing unusual. Here how we move them to BlogEngine application:
- All sample images go to new folder
~/app\_data/files/slider
. BlogEngine protects files, including images, by restricting access and allowing only known types and locations. App_data folder is one of locations you can access from application with image handler without issues. Also app_data/files is default location to store blog files and images you normally upload through admin panel. And file manager added after 2.5 release also let you manage files in app_data/files. So it makes lots of sense to put our custom images there. - jquery.nivo.slider.pack.js go to ~/scripts folder. Scripts is a "special" folder in BlogEngine - any JavaScript you drop in there will be automatically loaded at runtime and added to posts and pages. We might make it more efficient later and only add script to the page that actually has a slider on it, but lets make it work first.
- Style sheet nivo-slider.css and nivo-slider images folder with background images used in CSS all go under ~/styles. This is another special folder and it does the same thing for styles as "scripts" folder for JS.
- Hard-coded HTML markup and JavaScript call from HTML page go to ~/themes/standard/site.master. Because we moved images, all image paths need to be updated.
- Image source tags in HTML markup added to site.master now need to point to /app_data/files/slider. Because now we in server-side world, easiest way is to use "" with "runat=server" to let path be resolved dynamically. Here what markup looks like now:
<div id="slider" class="nivoSlider">
<a href="#"><img runat="server" src="~/image.axd?picture=Slider/sample1.png" alt="" /></a>
<a href=""> <img runat="server" src="~/image.axd?picture=Slider/sample2.png" title="sample two" alt="" /></a>
</div>
<script type="text/javascript">
$(window).load(function () { $('#slider').nivoSlider();});
</script>
Backgrounds in CSS need to point to ~/styles/nivo-slider. Because ~/themes/standard/site.master is the base page, all images can be accessed from styles using relative URL reaching two steps up: "../../styles/nivo-slider"
#slider { position:relative;background:url(../../Styles/nivo-slider/loading.gif) no-repeat 50% 40%; }
Here are files we used so far:
Scriptsjquery.nivo.slider.pack.js
Stylesnivo-slider *.*nivo-slider.css
themesStandard site.master
If you run plain vanilla BlogEngine.NET 2.5 with these changes in place, you'll get slider loaded and working as good as in HTML demo that comes with NivoSlider proper. Sure this is not very useful yet - you would need manually change markup in site.master each time you want to add or remove image from slide-show. But in the next step we'll build extension, user control and Razor helper that will make things lot more interesting and dynamic.