Using multiple settings in Extension Manager
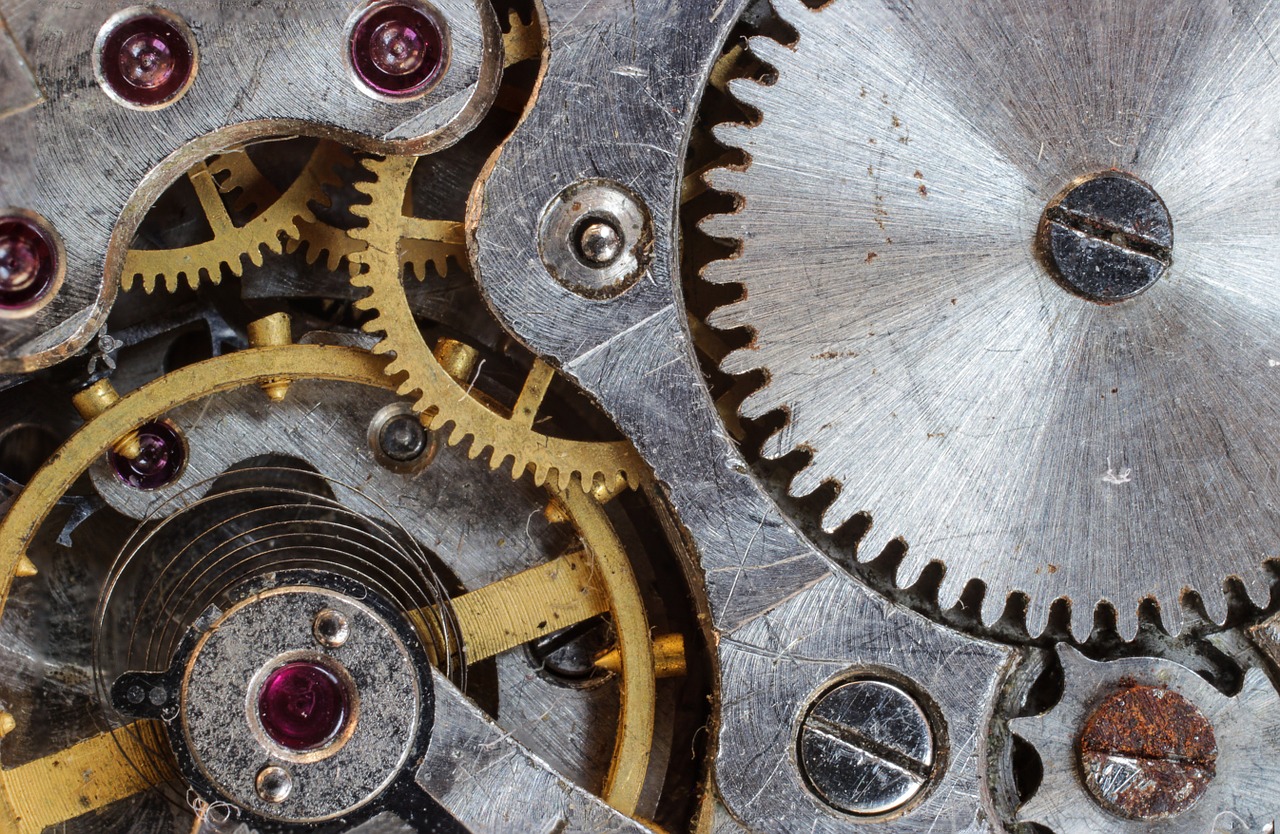
One of the limitations in the Extension Manager is that extension can handle only one settings object. In most cases, for simple extensions, that is enough. But sometimes you need to let blogger maintain multiple set of settings. Common scenario would be when you want to set some parameters and then maintain list of data. For example, in download counter I might want to have settings for kind of files or directories to watch for or ignore, and then I want to save download log into table structure. Currently – can’t do it without custom admin page.
The latest build will let you create extensions that can have multiple settings. There should be no changes to existing extensions, they will run just fine. To take advantage of multiple settings, all you need is to pass name of the extension along with the settings object that you want to save or retrieve. Here is a little example that should be self explanatory. I created little “Test” extension with two sets of settings, initialized them with default values and saved in the manager.
static protected ExtensionSettings _set1 = null;
static protected ExtensionSettings _set2 = null;
public Test()
{
ExtensionSettings set1 = new ExtensionSettings("The first setting");
set1.AddParameter("One");
set1.AddParameter("Two");
set1.IsScalar = true;
set1.AddValue("One", "10");
set1.AddValue("Two", "200");
ExtensionManager.ImportSettings("Test", set1);
ExtensionSettings set2 = new ExtensionSettings("My tabular setting");
set2.AddParameter("Code", "Code", 20, true);
set2.AddParameter("OpenTag", "Open Tag", 150, true);
set2.AddParameter("CloseTag", "Close Tag");
set2.Help = "Test for multiple settings per extension.";
set2.AddValues(new string[] { "b", "strong", "" });
set2.AddValues(new string[] { "i", "em", "" });
ExtensionManager.ImportSettings("Test", set2);
_set1 = ExtensionManager.GetSettings("Test", "The first setting");
_set2 = ExtensionManager.GetSettings("Test", "My tabular setting");
}
Now you can maintain values in the admin interface for both settings and easily get them in your client code:
// for scalar (flat) settings:
string s = _set1.GetSingleValue("One");
// or
Dictionary<string, string> dic = _set1.GetDictionary();
string d = dic["One"];
// for tabular data
DataTable table = _set2.GetDataTable();
You can bind controls to table or dictionary – they are standard .NET objects. Next natural step for me will be decoupling settings and manager, so that settings can be shared between different kind of plug-ins, like widgets, themes etc. This will let authors to write customizable themes and widgets without warring about how/where to store data for their components.