Strategy pattern
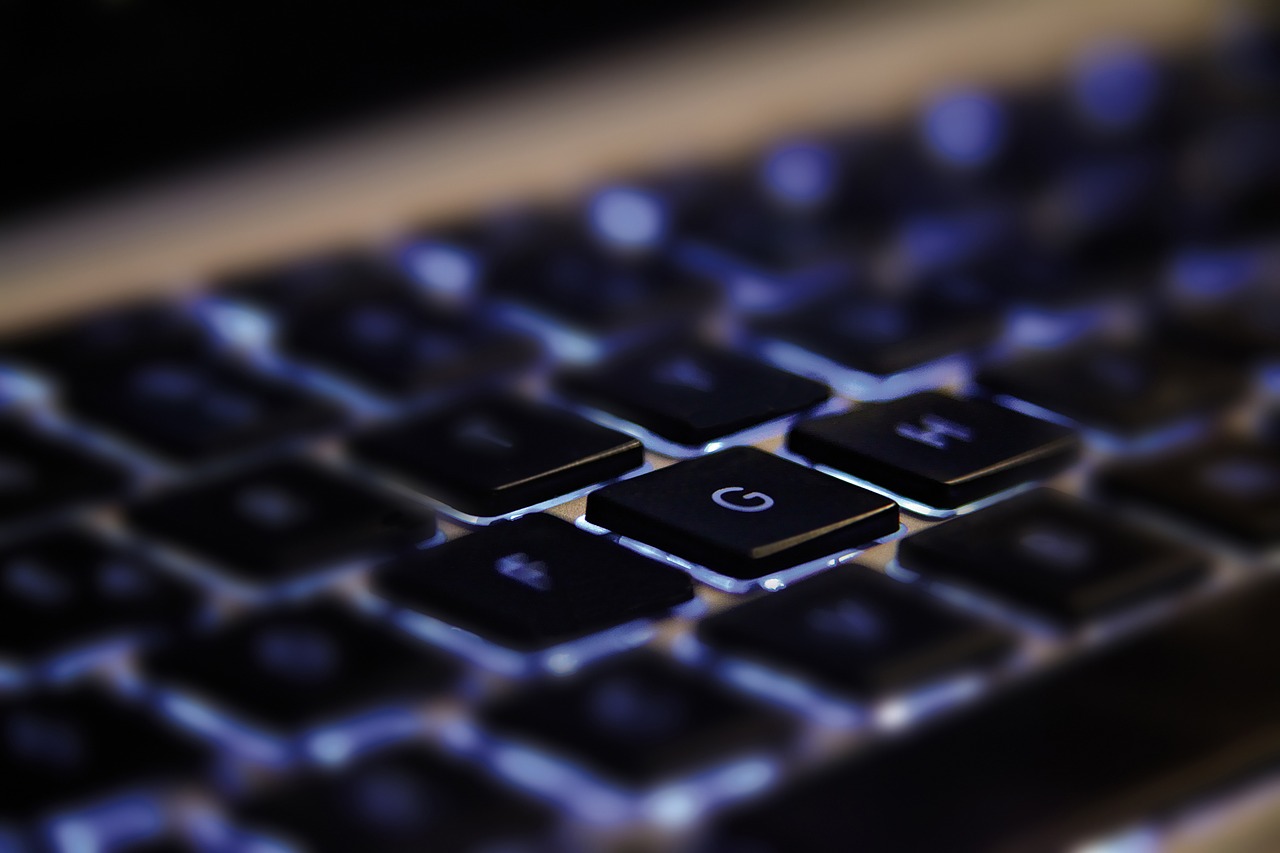
Do you use design patterns in your daily development? You probably should, and if you don't you might start with reading some books on the subject. I would suggest one from Head First series, although not everybody is a big fan of this book. But I found it fun and easy reading that can trigger your curiosity and encourage you to dig dipper. It is written for Java developers, but language samples presented in the book are minimal and, if you don't understand Java, you can refer to this project for C# translation.
Deign patterns are supposed to help us find solutions for common problems and in itself are no more than set of best practices applied for common scenarios. The usual wisdom is not to learn some cool pattern and use it right and left (solution looking for a problem approach) but rather use it as a bandage when you feel real pain. I got my pain trying to find a good way to handle differences in saving and retrieving settings for different types of extensions working on generic data store layer for BlogEngine 1.4 release. So I decided to look at some of the patterns to see if there is something applicable, and sure enough it turns out that I wasn't the first one dealing with this situation. Here is a strategy pattern as it is applied to Duck example in the very beginning of the book:
And here is my own, that gives me flexible and extendible way of building components that all kind of extensions can use to save and get back settings as different object types (on diagram, for simplicity, the "get" part is omitted). The obvious part is having base class that WidgetSettings and alike can inherit from. The meat of this pattern is to let you use behaviors - classes that encapsulate algorithms for specific purposes, in my case for saving different objects for different components.
Here how it works. In you client code (extension, widget or theme) you would create concrete class and use method to save your settings object:
WidgetSettings wset = new WidgetSettings();
wset.SaveSettings(Xml);
This class would set a behavior and simply delegate all hard work:
public class WidgetSettings : SettingsBase
{
public WidgetSettings()
{
base.ExType = ExtensionType.Widget;
SaveBehavior = new SaveXMLDocument();
}
}
And now that specialized behavior class will perform the real job - saving XML document or extension settings object to the database or file system. The beauty of this approach is that all things are nicely separated from each other and at the same time work well as a team.