Let Extension Manager manage settings
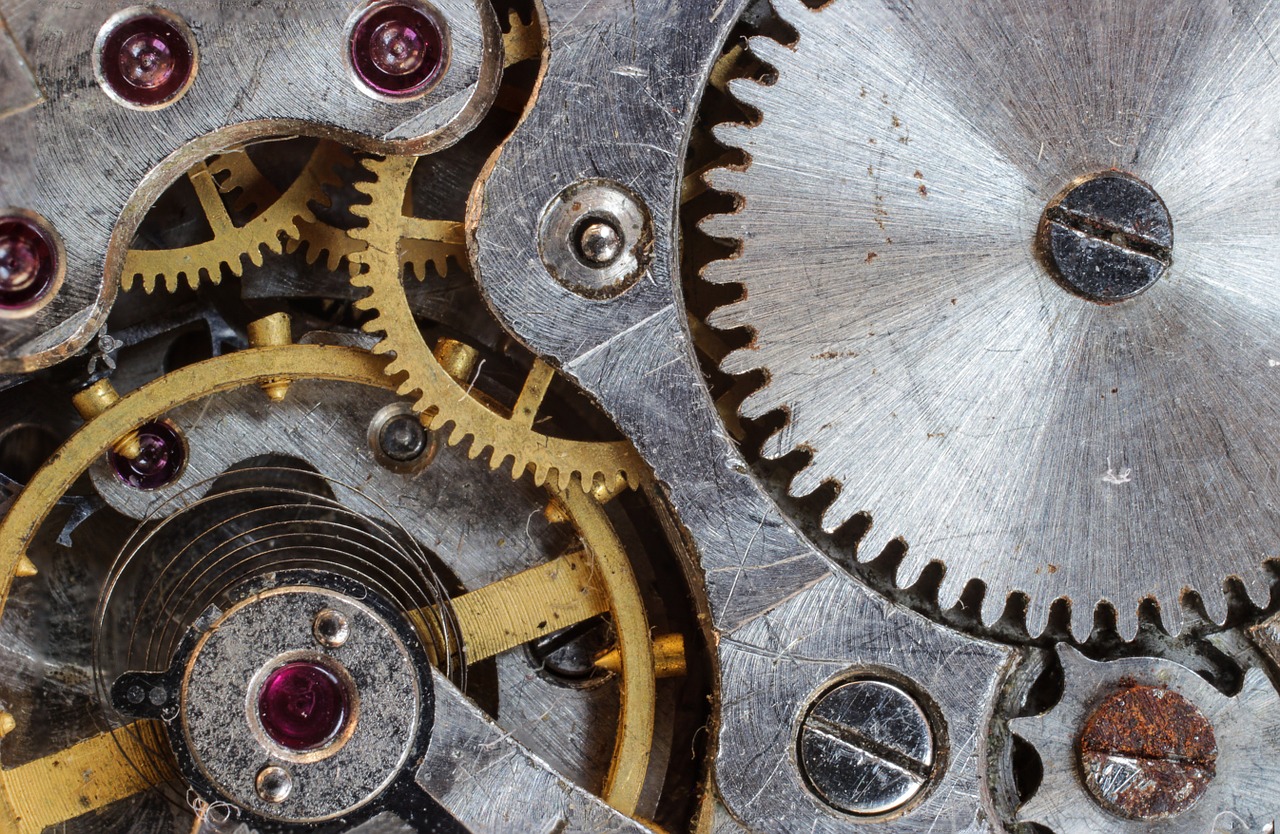
By now we have simple and functional admin interface for extension management. Let’s go beyond basics, how about default settings page that extension writers can take advantage of? Let me explain what I mean. Suppose, you wrote BBCode extension (hope Mads won’t be mad at me:). It let people use XHTML in their comments without compromising security. The way it works: you define a square bracket syntax that people can use and extension converts it into real markup on comment serve. This is what code looks like:
Parse(ref body, "b", "strong");
Parse(ref body, "i", "em");
Parse(ref body, "u", "span style=\"text-decoration:underline\"", "span");
Parse(ref body, "quote", "cite title=\"Quote\"", "cite");
If you need to add or remove things that people can use in their comments, you can go and modify these lines, adding or removing stuff. How many of you done so? Anybody? Well, it’s kind of a pain – especially when we talking hosting environment. This is a lot easier with extension like QuickLinks which comes with admin page:
But then, it is a pain for extension writer to implement admin page for simple extension. It will likely require more effort to do this than write actual extension. What if we put Extension Manager in charge of heavy lifting and let him manage settings? So, instead of implementing its own admin page, BBCode would have to:
- Declare settings as dictionary object.
- Try to load settings from cache (manager is responsible for populating it).
- If cache is empty, load dictionary with default values and cache it.
private Dictionary<string, string[]> Settings
{
get {
Dictionary<string, string[]> s = new Dictionary<string, string[]>();
if (HttpContext.Current.Cache["x:BBCode"] != null) {
s = (Dictionary<string, string[]>)HttpContext.Current.Cache["x:BBCode"];
}
else {
s.Add("b", new string[1]{"strong"});
s.Add("i", new string[1] {"em" });
s.Add("u", new string[2] {"span style=\"text-decoration:underline\"", "span"});
s.Add("quote", new string[2] { "cite title=\"Quote\"", "cite" });
HttpContext.Current.Cache["x:BBCode"] = s;
}
return s;
}
}
Use this dictionary object in your extension instead of hard-coded values.
private void Post_CommentServing(object sender, ServingEventArgs e)
{
string body = e.Body;
foreach (string key in Settings.Keys) {
string[] s = Settings[key];
if(s.Length == 2)
Parse(ref body, key, s[0], s[1]);
else
Parse(ref body, key, s[0]);
}
e.Body = body;
}
Extension and Manager communicate through the cache using common convention: cache key should be named as “x:extensionname” and must contain a dictionary object. If this is true, extension and manager have shared storage and can use it to pass dada to each other. This way you don’t have to implement your own admin interface (which over time probably would require admin panel re-design to handle many more additional tabs) and all extension admin pages are neatly lined up under common UI and easy to use. Everybody wins!