Writing extensions for BlogEngine 1.4 (part 1)
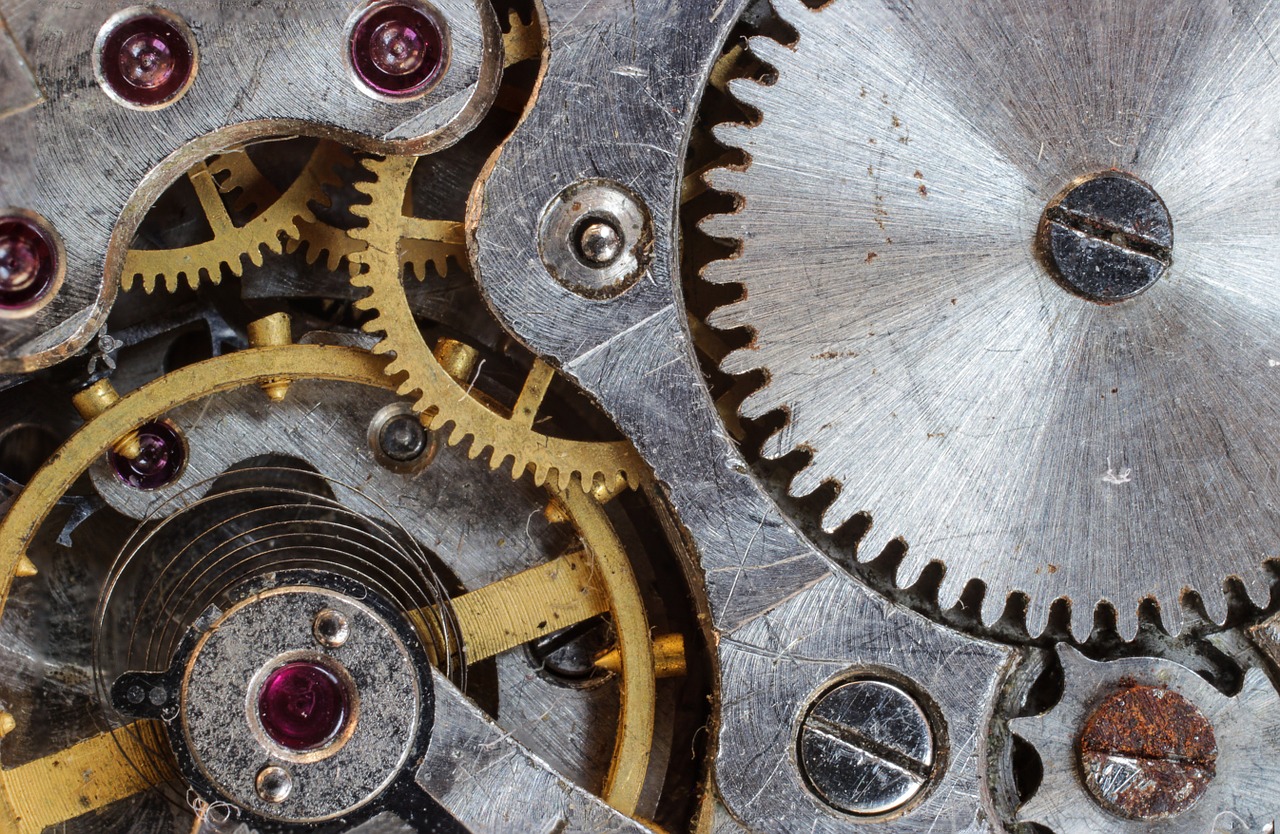
This is the first part in the series of tutorials about writing extensions for the BlogEngine 1.4. I’ll start with simple “hello world” example and then gradually move to the more advanced techniques. You don’t need to have any previous experience with BE extensions to follow this tutorial.
BlogEngine extension is just a class file that you can drop in the App_Code folder. That means, you can use any editor to write it, from Notepad to full blown Visual Studio. Express edition also works fine. For VS, you can start a new class library, set reference to BlogEngine.Core.Dll and you’ll get all VS functionality enabled. Obviously, you can download complete source code from CodePlex repository and use it as your project, if fact this is a preferable method as you’ll be able to brows files easier. You can use VS 2005 or convert solution to VS 2008. Whatever your choice is, create a new file, call it Example_01.cs then copy and paste code shown below.
using System;
using BlogEngine.Core.Web.Controls;
using BlogEngine.Core;
[Extension("Example_01", "1.0", "Me")]
public class Example_01
{
public Example_01()
{
Post.Serving += new EventHandler<ServingEventArgs>(ServingHandler);
}
private void ServingHandler(object sender, ServingEventArgs e)
{
e.Body = e.Body.ToLower();
}
}
This example is very simple, let’s go over it to understand what it is doing. First of all, we need to import Core libraries to get access to BE objects like Post, Page etc. with “using” statement. Then, we need to decorate our class with “Extension” attribute. BlogEngine will examine class attributes on application start up and, if class is marked as extension, it will create instance of this class and hook up the event handlers which is vital for extension to work. Attribute has three properties: extension name, version, and author. Extension must have a default public constructor without parameters, this is where we subscribe to Post.Serving event (list of events can be found here). Now, every time this event fired (Post is served to the web browser) function ServingHandler will be executed.
public static void OnServing(Post post, ServingEventArgs arg)
This function takes two arguments: Post and ServingEventArgs. ServingEventArgs has two important properties: Body, the string representing content of the post, and Location. Location can tell you what kind of post been served. When you use Post.Serving it is either PostList or SinglePost. Body gives you access to content of the post, so you can easily manipulate it. In this example, we change post content to lower case. Copy Example_01.cs to the App_Code folder in the working BlogEngine web site and go to the front page. IIS will automatically restart when it finds new class in this folder, reloads application and extension will become part of the web site. When page is loaded, you should see each post in lower case. Not very useful at this point yet, but as you can see it is very easy to write and use BE extension.
If you click Extensions link under Administration menu, it will bring you to the list of all extensions used by your blog. The new extension, Example_01, will be listed here too. Version, description and author taken straight from the extension attribute in the class we just created. You can enable or disable extension (you’ll need ASP.NET user have write permissions on App_Code folder for this to work). You can also look at extension’s source code if you click “View” link under Source column in the list. Our simple extension does not have settings, so you don’t see “Edit” link next to the extension name.
In the next tutorial we examine what extension settings are, why you (often) need them and how to use Extension Manager to manage extensions and settings in the BlogEngine 1.4.