Writing extensions for BlogEngine 1.4 (part 2)
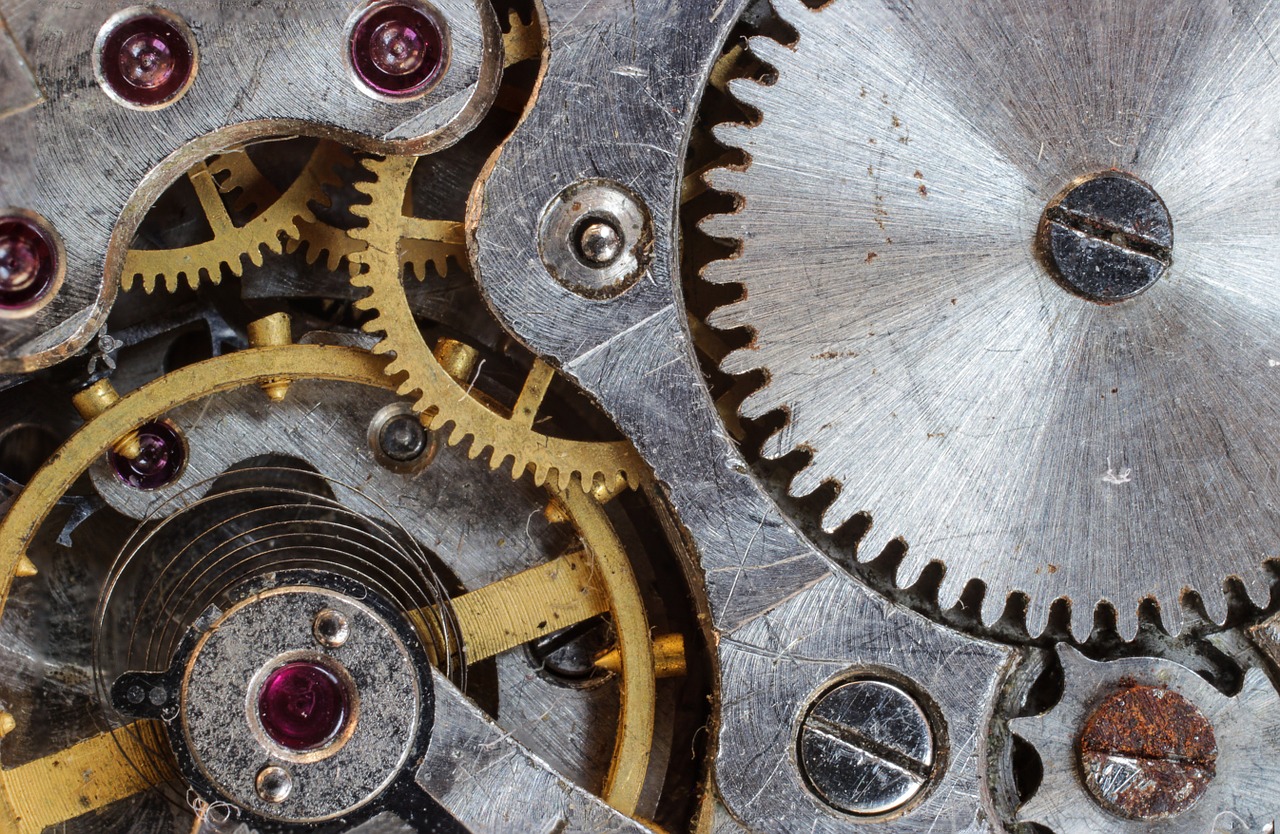
In the first part, we wrote simple extension that changes case in the post to lower. Let’s say, we want user to decide show post in the lower or upper case. For this, we need to be able to maintain variable and let blogger change it’s value through admin interface. Normally, you would need to add a data access functionality for extension to handle this kind of operation, create admin form etc. BlogEngine uses Extension Manager to help make job a lot easier. Take a look at the code below. In the constructor, we subscribe to post serving event and then call InitSettings. There we create ExtensionSettings object, which can hold singe value (scalar) or/and table of values. In our case, we want it to hold single scalar value, so we set settings to scalar. We call our variable “Case” and initialize it with default value of “upper”, then call ExtensionManager.InitSettings and pass name of extension and settings object.
using System;
using BlogEngine.Core.Web.Controls;
using BlogEngine.Core;
[Extension("Example_02", "1.0", "<a href=\"http://me.net\">Me</a>")]
public class Example_02
{
static protected ExtensionSettings _settings = null;
public Example_02()
{
Post.Serving += new EventHandler<ServingEventArgs>(ServingHandler);
InitSettings();
}
private void ServingHandler(object sender, ServingEventArgs e)
{
if (_settings.GetSingleValue("Case") == "upper")
e.Body = e.Body.ToUpper();
else
e.Body = e.Body.ToLower();
}
private void InitSettings()
{
ExtensionSettings settings = new ExtensionSettings(this);
settings.IsScalar = true;
settings.AddParameter("Case");
settings.AddValue("Case", "upper");
_settings = ExtensionManager.InitSettings("Example_02", settings);
}
}
If you go to the settings list, you’ll see a link to the settings object next to the extension name. Clicking it will bring the screen shown below. Here blogger can maintain variable that we can use in the extension to determine what case to apply to the post. And this is what ServingHandler function does: it uses settings object saved during instantiation to get value of the “Case” parameter and, if it has value “upper” – set post to upper, else – set to lower. When value changed, it will be saved either in the file system or in the database – depending on the provider that blog uses.
The case variable was a string, but there are other data typed we could use. The Example_03 shows which types are supported by BlogEngine 1.4.
using System;
using BlogEngine.Core.Web.Controls;
using BlogEngine.Core;
using System.Collections.Generic;
using System.Collections.Specialized;
[Extension("Example_03", "1.0", "<a href=\"http://me.net\">Me</a>")]
public class Example_03
{
static protected ExtensionSettings _settings = null;
public Example_03()
{
Comment.Serving += new EventHandler<ServingEventArgs>(Post_CommentServing);
InitSettings();
}
private void Post_CommentServing(object sender, ServingEventArgs e)
{
Comment comment = (Comment)sender;
if (comment.Author != "typetest")
return;
foreach (ExtensionParameter p in _settings.Parameters)
{
if (string.IsNullOrEmpty(p.SelectedValue))
e.Body += "<br/>" + p.Name + ": " + p.Values[0];
else
e.Body += "<br/>" + p.Name + ": " + p.SelectedValue;
}
}
private void InitSettings()
{
ExtensionSettings settings = new ExtensionSettings(this);
settings.IsScalar = true;
// define parameters
settings.AddParameter("TheString");
settings.AddParameter("TheBoolean", "The boolean");
settings.AddParameter("TheInteger", "The integer", 10);
settings.AddParameter("TheLong", "The Long", 20, false);
settings.AddParameter("TheFloat", "The Float", 10, false, false, ParameterType.Float);
settings.AddParameter("TheDouble", "The Double", 15, false, false, ParameterType.Double);
settings.AddParameter("TheDecimal", "The Decimal", 20, false, false, ParameterType.Decimal);
// lists
settings.AddParameter("TheDropdown");
settings.AddParameter("TheListBox", "The ListBox", 20, false, false, ParameterType.ListBox);
settings.AddParameter("TheRadioGroup", "The RadioGroup", 20, false, false, ParameterType.RadioGroup);
// set default values
settings.AddValue("TheString", "Test string");
settings.AddValue("TheBoolean", true);
settings.AddValue("TheInteger", 25);
settings.AddValue("TheLong", 9223372036854);
settings.AddValue("TheFloat", 25.7);
settings.AddValue("TheDouble", 523456789.35);
settings.AddValue("TheDecimal", decimal.Parse("9223372036854342342.345"));
// lists
StringCollection dd = new StringCollection();
dd.AddRange(new string[] { "One", "Two", "Three" });
settings.AddValue("TheDropdown", dd, "Three");
settings.AddValue("TheListBox", new string[] { "List1", "List2", "List3" }, "List2");
settings.AddValue("TheRadioGroup", new string[] { "Radio1", "Radio2", "Radio3" }, "Radio1");
_settings = ExtensionManager.InitSettings(this.GetType().Name, settings);
}
}
As you can see from the example and the picture, there are standard types like integer, decimal etc. Boolean, as one would expect, rendered as check box. There are also List data types. Lists render on the admin form in three different ways: as a drop down, as list box and as radio button group. There is standard type checking – if you try to save not integer value in the parameter declared as integer, you’ll get an error message. Check Exemple_03 code on how to declare different types of parameters. Just note that if you not specify parameter type and pass to AddParameter, say, integer – BE will check parameter type and make a best guess, assigning integer, decimal etc. based on variable you passed as default value.
This example uses comment serving event, which is fired when comment is served. For the purpose of showing how to get values back from settings, event handler outputs each parameter and its value in the comment body – if commenter is “typetest”. In the standard theme, you can switch to preview to see what it looks like.
To get strongly typed value, use conversion function. For example:
int i = int.Parse(p.Values[0]);
Next time I’ll go over Extension Manager and ways of using it in BlogEngine 1.4.